Ghost Shot
3D Platformer
Video created and voiced by me.
A cozy 3D platformer set in a sandbox of supernatural abilities. Hop across the land on a journey to photograph ghosts and set them free.
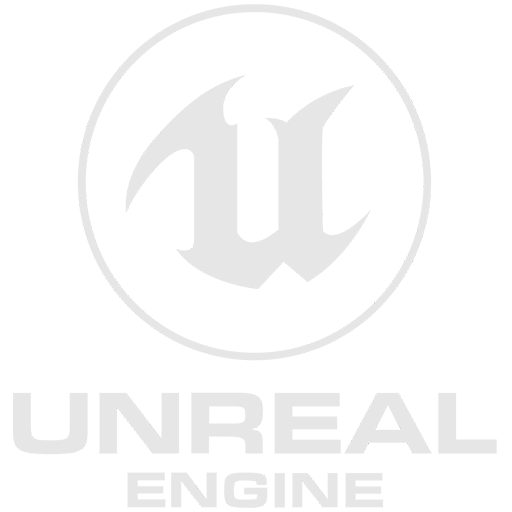
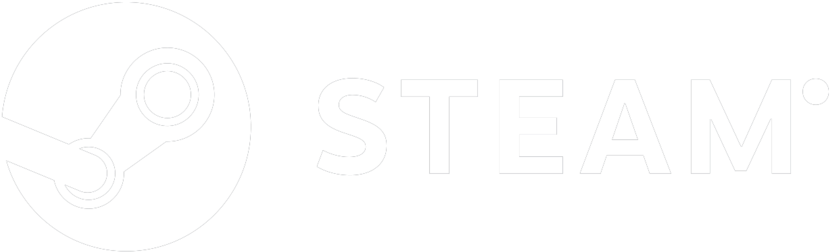
Halloween 2024
Responsive 3D Movement
Problem Statement
Fluid character movement is the cornerstone of effective platformer design.
Player Experience Goals
-
Predict the Player
Buffer inputs and logically blend between actions to make movement feel effortless.
-
Provide Clear Feedback
Movement should provide visual and audio cues to improve the player's sense of control.
-
Achieve Low Input Latency
Translate player input immediately into visible action, decreasing the friction between themselves and the game experience.
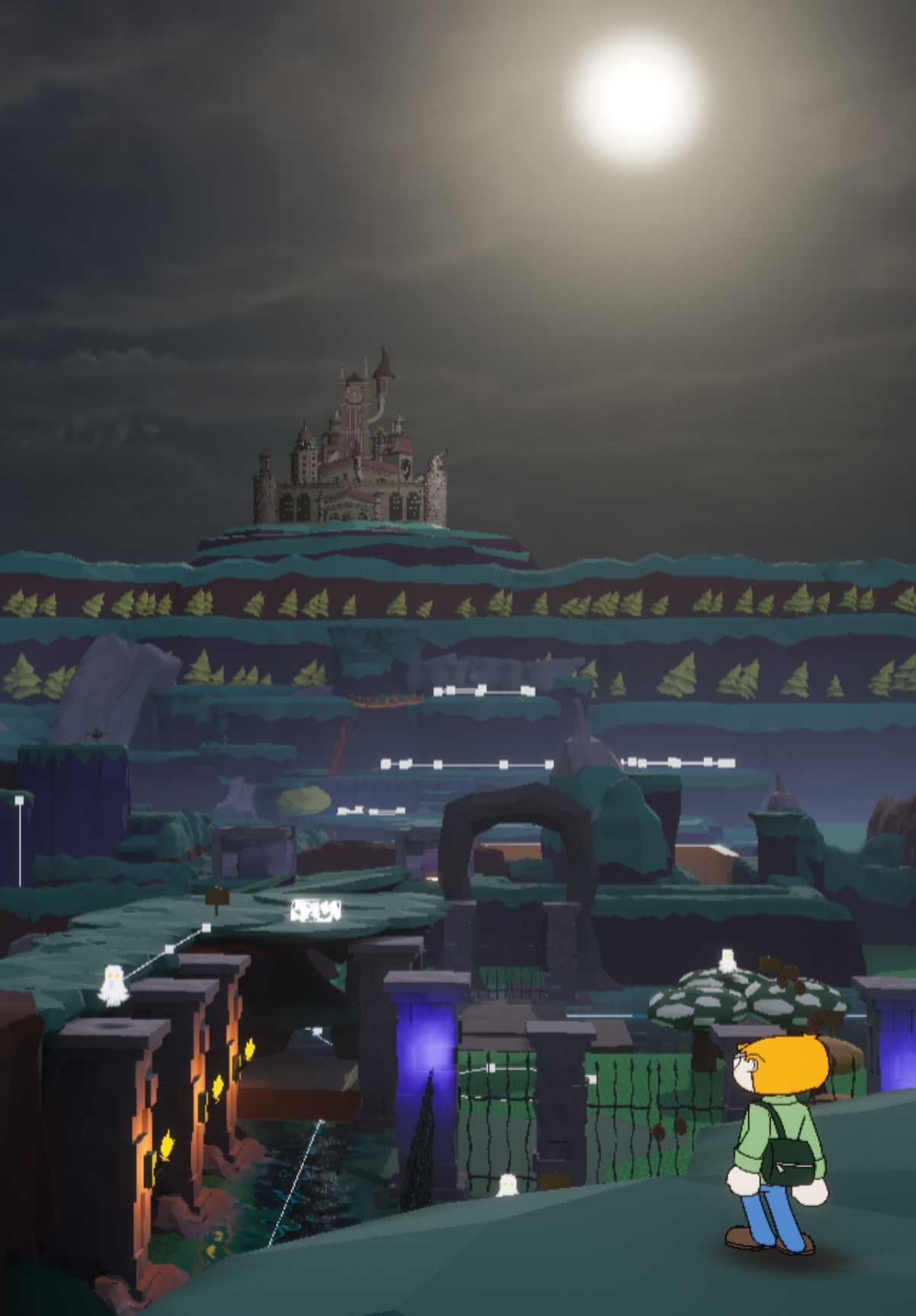
I designed a number of responsive systems that increase the level of control the player has over the character.
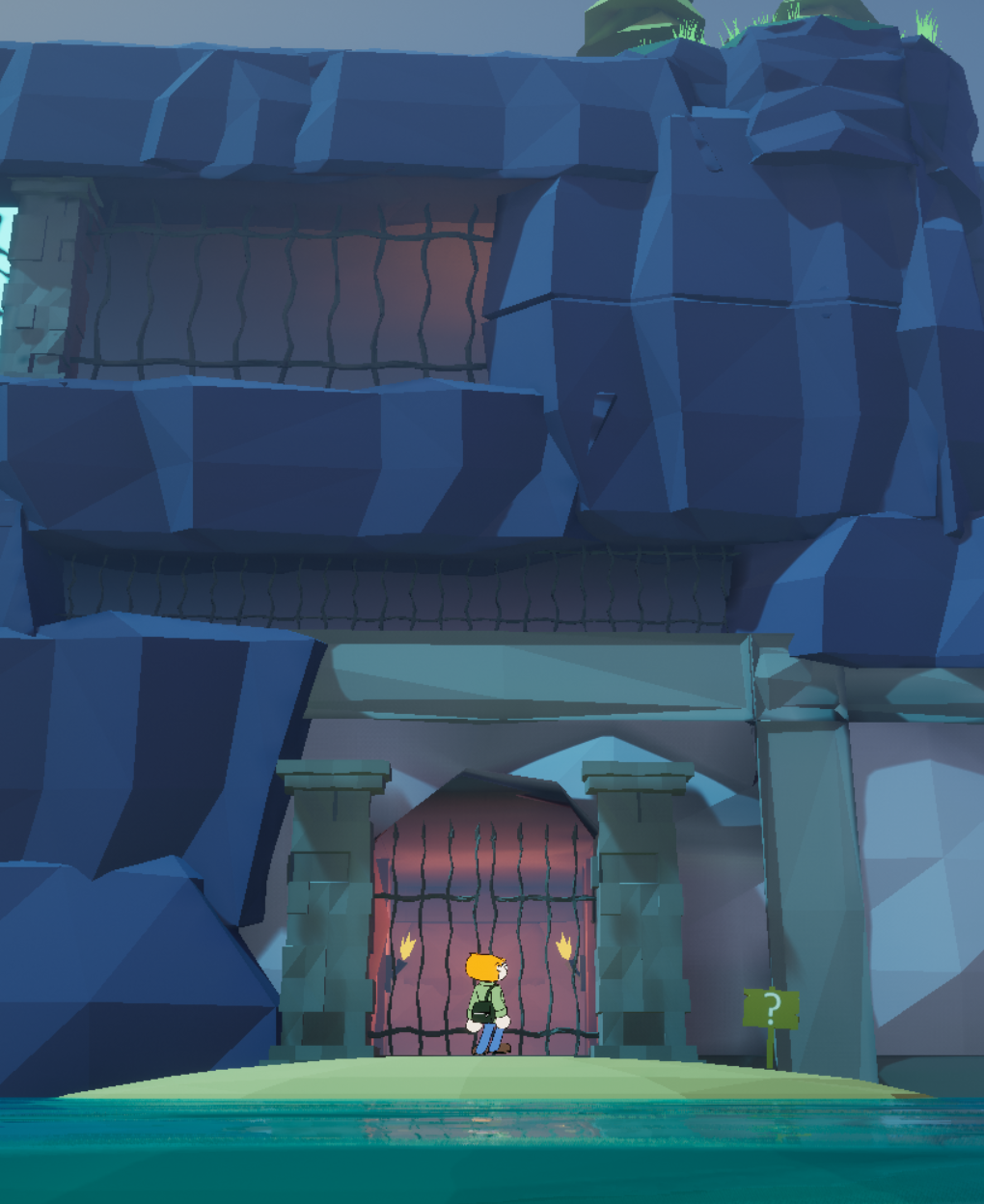
A key challenge for me while designing movement — our character is 2D!
How can we effectively convey the direction the player is running in, the speed of the character, and more in 3D space with only a 2D sprite?
I used a variety of visual cues paired with audio to convey the character’s movement state to the player, allowing for strong input feedback despite using a flat sprite.
This contributed greatly to a sense of decreased input latency and increased control over the character.
Designing a Good Jump
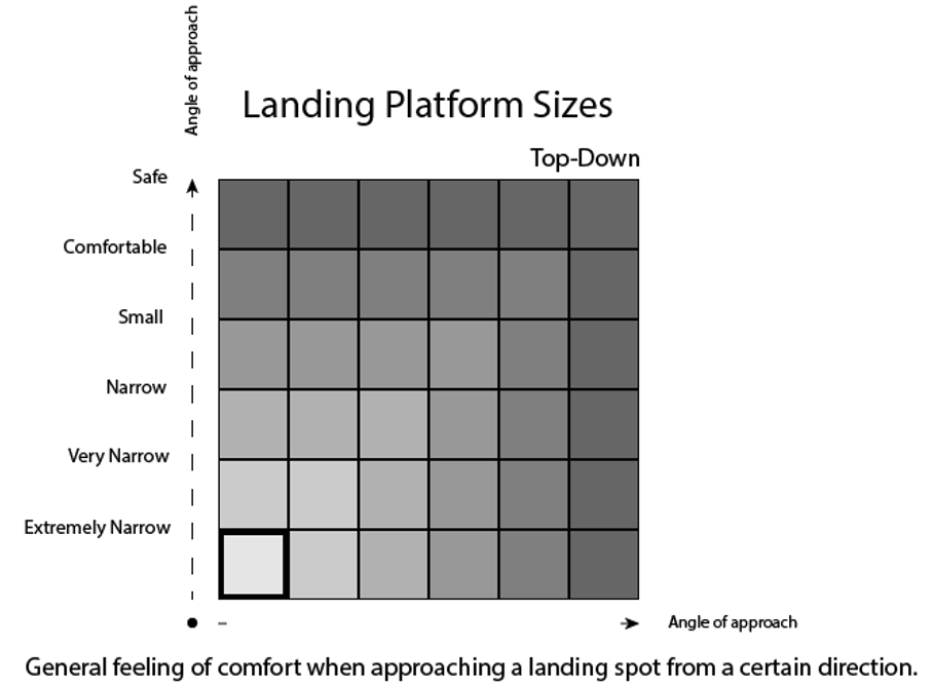
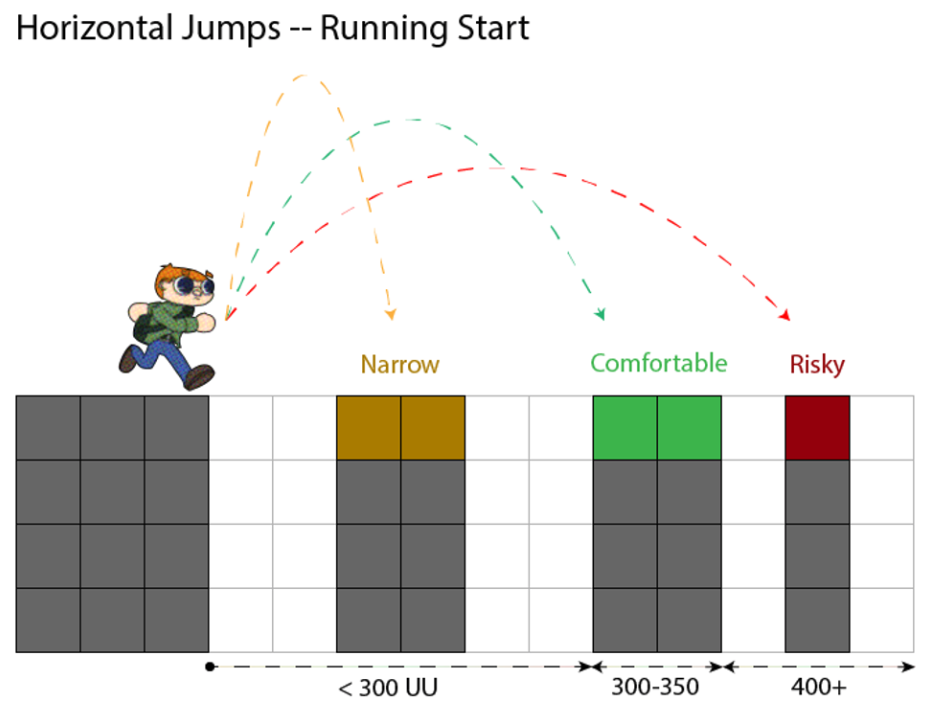
Level design plays a large role in how a game feels to play. Jumping is the most common action a player will do, and I therefore wanted it to feel as good as possible.
An early playtest finding was that the amount of space available for landing on a platform influences the intensity of the challenge.
This diagram is scaled to 50 Unreal Units Squared per tile, and was used as reference for designing platforming challenges.
The movement system I programmed halves gravity at the peak of a jump, which means that the jump apex is when the player has the most control. From playtest feedback and iteration, I determined that the ideal jump occurs when the player character is at the apex of their jump right before landing on a platform.
All of our jumps follow this principle. We modify the landing sizes to vary intensity and design each jump to coincide with where a player will reach the apex just as they arrive over a platform.
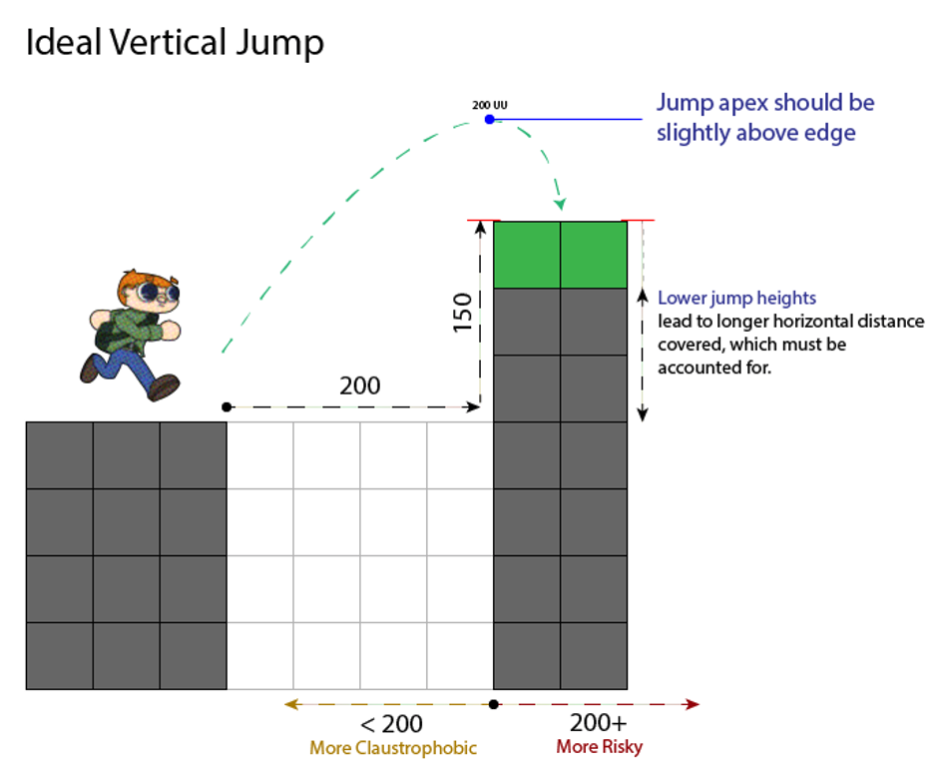
Designing Effortless Controls
Our user experience research revealed that the bottom and left face buttons (A and X), the right trigger, and the left thumbstick are the most easy to reach buttons on a standard controller.
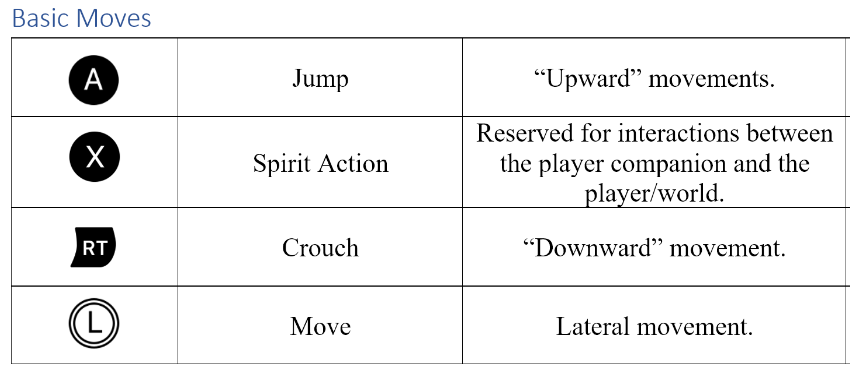
I committed to using those buttons as the primary movement buttons, each with its own role. This ensured general movement was as comfortable as possible on a controller.
From there, I developed an advanced moveset that used combinations of the primary movement buttons. This allowed for more movement possibilities, such as spinning in midair, diving, crawling, and more.
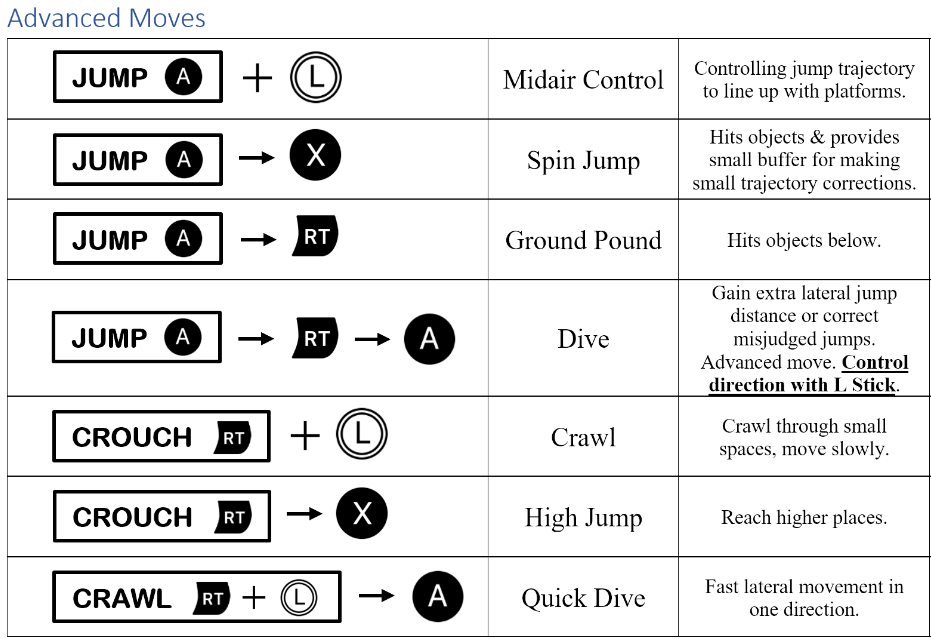
Additionally, some buttons, such as jump, provided more of that input the longer they were held down.
With just the four most reachable controller buttons, I was able to achieve an expressive moveset that combines each move in meaningful ways.
In our playtesting, this drastically increased the ease of control the player had. More importantly, the complex moveset removed friction between movement states (jumping, walking, etc.), allowing players to seamlessly transition into the next move they wanted to execute.
C++ Gameplay Framework
Engineering Approach
My approach to gameplay programming was to build or refactor all core gameplay functionality as native C++ code. This code is designed to be extended in Blueprint to allow for rapid prototyping.
To accomplish this, code needed to be written in a way that made it easy to compose by calling functions exposed to Blueprints.
Engineering Goals
-
Extensibility
Code built to be extended with new functionality, including new mechanics and prototypes.
-
Performance
Code that is cache-coherent and uses the minimal amount of clock cycles.
-
Good Documentation
Good comments ensure other members of the team can work productively with the codebase.
Player Character Class
The player character is made up of a core C++ class (extends APawn), and then composed of multiple actor components that each encapsulate some functionality.
For example, player movement and input handling is done by the PlayerMovementController class. The player’s graphical representation and visual effects is done in PlayerGraphicsController.
This approach allows programmers on the team to reason about encapsulated chunks of functionality, rather than deal with the complexity of the entire character class and everything it does.
Additionally, this also allows for interchangeability among components. Components are not dependent or aware of each other by design, and communicate via event dispatchers in the base character class. This allows each component to be extended, rewritten, or redone to provide new functionality without rewriting other parts of the character class.
// Copyright 2022 Soup Initiative Games #include "ProjectSpooky64/Public/Pawns/PlayerMovementController.h" #include "ProjectSpooky64/Public/Pawns/APlayer_Character.h" #include "PaperCharacter.h" #include "Components/CapsuleComponent.h" #include "GameFramework/Actor.h" #include "Kismet/KismetMathLibrary.h" #include "Kismet/GameplayStatics.h" // Sets default values for this component's properties UPlayerMovementController::UPlayerMovementController() { // Set this component to be initialized when the game starts, and to be ticked every frame. You can turn these features // off to improve performance if you don't need them. PrimaryComponentTick.bCanEverTick = true; // ... MoveState = EMoveState::Idle; MoveSet = EMoveSet::Standard; } // Called when the game starts void UPlayerMovementController::BeginPlay() { Super::BeginPlay(); //Ref_CharacterMovement = GetOwner()->FindComponentByClass<UCharacterMovementComponent>(); Parent = Cast<AAPlayer_Character>(GetOwner()); Ref_CharacterMovement = Parent->GetCharacterMovement(); Parent->JumpMaxHoldTime = JumpBufferMaxTime; Parent->OnMoveStateChanged.AddDynamic(this, &UPlayerMovementController::NotifyStateChanged); Parent->OnMoveSetChanged.AddDynamic(this, &UPlayerMovementController::NotifySetChanged); Parent->OnLanded.AddDynamic(this, &UPlayerMovementController::NotifyOnLanded); Parent->OnJumped.AddDynamic(this, &UPlayerMovementController::NotifyOnJumped); Parent->OnLaunched.AddDynamic(this, &UPlayerMovementController::NotifyOnLaunched); Parent->HitMechanic.AddDynamic(this, &UPlayerMovementController::NotifyHitMechanicFired); Parent->CameraMechanic.AddDynamic(this, &UPlayerMovementController::NotifyCameraMechanicFired); StateFunction = &UPlayerMovementController::State_Idle; (this->*StateFunction)(EStateInput::INIT); } // Called every frame void UPlayerMovementController::TickComponent(float DeltaTime, ELevelTick TickType, FActorComponentTickFunction* ThisTickFunction) { Super::TickComponent(DeltaTime, TickType, ThisTickFunction); //UE_LOG(LogTemp, Warning, TEXT("Jump buffered: %s"), (JumpInputBuffered) ? TEXT("TRUE") : TEXT("false")); } void UPlayerMovementController::GuardedTick_Implementation(float DeltaTime) { _Internal_DeltaTime = DeltaTime; //_SetInputVectorAndRotation(); // todo: might be able to move this to a lower function later _CalculateInputRotationFromX(); (this->*StateFunction)(EStateInput::TICK); //UE_LOG(LogTemp, Warning, TEXT("Can the parent jump: %s"), (Parent->CanJump() ? TEXT("true") : TEXT("false"))); //_Debug_PrintState(); } float UPlayerMovementController::GetInternalDeltaTime() { return _Internal_DeltaTime; } void UPlayerMovementController::SetMoveState(EMoveState NewState) { MoveState = NewState; } void UPlayerMovementController::SetMoveSet(EMoveSet NewSet) { MoveSet = NewSet; } EMoveState UPlayerMovementController::GetMoveState() { return MoveState.GetValue(); } EMoveSet UPlayerMovementController::GetMoveSet() { return MoveSet.GetValue(); } void UPlayerMovementController::InitializeInputs(UInputComponent* PlayerInputComponent) { UE_LOG(LogTemp, Warning, TEXT("PlayerMovementController initialized.")); PlayerInputComponent->BindAction("Jump", EInputEvent::IE_Pressed, this, &UPlayerMovementController::JumpActionPressed); PlayerInputComponent->BindAction("Jump", EInputEvent::IE_Released, this, &UPlayerMovementController::JumpActionReleased); PlayerInputComponent->BindAction("Crouch", EInputEvent::IE_Pressed, this, &UPlayerMovementController::CrouchActionPressed); PlayerInputComponent->BindAction("Crouch", EInputEvent::IE_Released, this, &UPlayerMovementController::CrouchActionReleased); PlayerInputComponent->BindAction("Flash", EInputEvent::IE_Pressed, this, &UPlayerMovementController::FlashActionPressed); PlayerInputComponent->BindAction("Flash", EInputEvent::IE_Released, this, &UPlayerMovementController::FlashActionReleased); PlayerInputComponent->BindAction("Grab", EInputEvent::IE_Pressed, this, &UPlayerMovementController::GrabActionPressed); PlayerInputComponent->BindAction("Grab", EInputEvent::IE_Released, this, &UPlayerMovementController::GrabActionReleased); PlayerInputComponent->BindAction("Hit", EInputEvent::IE_Pressed, this, &UPlayerMovementController::HitActionPressed); PlayerInputComponent->BindAction("Hit", EInputEvent::IE_Released, this, &UPlayerMovementController::HitActionReleased); PlayerInputComponent->BindAxis("HorizontalMovement", this, &UPlayerMovementController::HorizontalAxisInput); PlayerInputComponent->BindAxis("VerticalMovement", this, &UPlayerMovementController::VerticalAxisInput); } void UPlayerMovementController::JumpActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::JUMP_Pressed); } void UPlayerMovementController::JumpActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::JUMP_Released); } void UPlayerMovementController::CrouchActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::CROUCH_Pressed); //UE_LOG(LogTemp, Warning, TEXT("Crouch action pressed")); } void UPlayerMovementController::CrouchActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::CROUCH_Released); } void UPlayerMovementController::FlashActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::FLASH_Pressed); } void UPlayerMovementController::FlashActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::FLASH_Released); Parent->ReleaseCameraMechanic(); } void UPlayerMovementController::GrabActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::GRAB_Pressed); Parent->FireAimMechanic(); // Might want to refactor into a state helper function. UE_LOG(LogTemp, Warning, TEXT("Grab action pressed")); } void UPlayerMovementController::GrabActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::GRAB_Released); Parent->ReleaseAimMechanic(); // Might want to refactor into a state helper function. UE_LOG(LogTemp, Warning, TEXT("Grab action released")); } void UPlayerMovementController::HitActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::HIT_Pressed); } void UPlayerMovementController::HitActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::HIT_Released); Parent->ReleaseHitMechanic(); } void UPlayerMovementController::HorizontalAxisInput(float AxisValue) { } void UPlayerMovementController::VerticalAxisInput(float AxisValue) { } void UPlayerMovementController::SetMoveEnabled(bool Enabled) { if (Enabled) { this->InputEnabled = true; Parent->_SetMovementInputEnabled(true); } else { this->InputEnabled = false; Parent->_SetMovementInputEnabled(false); ResetMovement(); } } float UPlayerMovementController::GetHorizontalAxisValue() { if (!InputEnabled) { return 0.f; } return Parent->GetInputAxisValue("HorizontalMovement"); } float UPlayerMovementController::GetVerticalAxisValue() { if (!InputEnabled) { return 0.f; } return Parent->GetInputAxisValue("VerticalMovement"); } void UPlayerMovementController::ResetMovement() { (this->*StateFunction)(EStateInput::RESET); NewState(EMoveState::Idle); Ref_CharacterMovement->Velocity = FVector{ 0.f, 0.f, 0.f }; } void UPlayerMovementController::State_Idle_Implementation(EStateInput input) { switch(MoveSet) { case Limited: Limited_Idle(input); break; default: Standard_Idle(input); break; } } void UPlayerMovementController::State_Run_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Run(input); break; default: Standard_Run(input); break; } } void UPlayerMovementController::State_Jump_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Jump(input); break; default: Standard_Jump(input); break; } } void UPlayerMovementController::State_HighJump_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_HighJump(input); break; } } void UPlayerMovementController::State_SpinJump_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_SpinJump(input); break; } } void UPlayerMovementController::State_Fall_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Fall(input); break; default: Standard_Fall(input); break; } } void UPlayerMovementController::State_Crouch_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Crouch(input); break; default: Standard_Crouch(input); break; } } void UPlayerMovementController::State_Crawl_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Crawl(input); break; default: Standard_Crawl(input); break; } } void UPlayerMovementController::State_Pound_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_Pound(input); break; } } void UPlayerMovementController::State_PoundFlip_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_PoundFlip(input); break; } } void UPlayerMovementController::State_Dive_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_Dive(input); break; } } void UPlayerMovementController::State_Dodge_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_Dodge(input); break; } } /* * Note: * When extending in Blueprint, * call Super/parent version for every case that isn't going to have a unique Blueprint implementation. */ void UPlayerMovementController::Standard_Idle_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Idle); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (NormalMovement()) // If movement is being applied { NewState(EMoveState::Running); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crouching); return; case HIT_Pressed: // fire companion hit (prob in parent APlayer_Character) break; case END: break; default: break; } } void UPlayerMovementController::Standard_Run_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Running); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (!NormalMovement()) { NewState(EMoveState::Idle); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crawling); break; default: break; } } void UPlayerMovementController::Standard_Jump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } /* * Returns true if NewState is called in CommonJumpAction's scope, therefore preventing the remainder of this function * from firing after the state has been changed. */ if (CommonJumpActions(input)) { return; } if (_RegularJump(input)) { return; } if (_JumpTransitionGuard(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Jumping); //UE_LOG(LogTemp, Warning, TEXT("Jump max count: %i"), Parent->JumpMaxCount); //Parent->Jump(); //CharMovement_JumpBuffered = true; // Guard for notifying TICK case that there is a jump waiting to be applied next update if (!Parent->CanJump()) { NewState(EMoveState::Idle); return; } break; case TICK: //UE_LOG(LogTemp, Warning, TEXT("Jump buffered: %s"), (JumpInputBuffered) ? TEXT("TRUE") : TEXT("false")); if (!Ref_CharacterMovement->IsFalling() && (!JumpInputBuffered || !Parent->CanJump())) //todo: can you refactor this to EVENT_Landed? { NewState(EMoveState::Idle); return; } /*todo: if jump is called but cannot be processed, call idle*/ //CharMovement_JumpBuffered = false; break; case JUMP_Released: Parent->StopJumping(); break; case CROUCH_Released: break; case EVENT_Landed: Parent->StopJumping(); break; case EVENT_Jumped: //CharMovement_JumpBuffered = false; break; case END: //CharMovement_JumpBuffered = true; //Parent->JumpMaxCount = 1; break; default: break; } } bool UPlayerMovementController::_RegularJump(EStateInput input) { switch (input) { case INIT: Parent->Jump(); break; case END: Parent->JumpMaxCount = 1; break; default: break; } return false; } bool UPlayerMovementController::CommonOnLandedActions_Implementation(EStateInput input) { switch (input) { case RESET: case EVENT_Landed: SpinJumpsAttempted = 0; DivesAttempted = 0; Parent->StopJumping(); break; default: break; } return false; } void UPlayerMovementController::Standard_HighJump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (CommonJumpActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::HighJumping); Parent->LaunchCharacter(FVector(0.f, 0.f, Ref_CharacterMovement->JumpZVelocity * 1.75), true, true); break; case TICK: break; case EVENT_Landed: //todo: is this going to cause errors? revisit if errors occur around highjumping NewState(EMoveState::Idle); return; default: break; } } void UPlayerMovementController::Standard_SpinJump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } switch (input) { case INIT: { SpinJumpsAttempted++; Parent->_SetMoveState(EMoveState::SpinJumping); __DefaultGravityScalePeak = GravityScalePeak; // Note: Alters state variables here, which are reset once this state ends. GravityScalePeak = GravityScalePeak / 2.f; FVector _Velocity = Ref_CharacterMovement->Velocity; FVector2D F2D_Velocity{ _Velocity.X, _Velocity.Y }; /* FVector InputVector = InputRotationFromX.Vector(); // get input from player forward vector FVector2D F2D_InputVector{ InputVector.X, InputVector.Y }; // make 2D F2D_Velocity = F2D_Velocity * F2D_InputVector; // @todo: this should redirect velocity in direction of movement */ FVector2D F2D_VelocityDirection{ 0.f }; float F2D_VelocityMagnitude = 0.f; // we want the magnitude F2D_Velocity.ToDirectionAndLength(F2D_VelocityDirection, F2D_VelocityMagnitude); FVector2D F2D_InputVector{ RawInputVector.X, RawInputVector.Y }; // get raw input vector 2D FVector InputVect = InputRotationFromX.Vector(); F2D_Velocity.X = InputVect.X; F2D_Velocity.Y = InputVect.Y; if (Parent->IsApplyingInput()) // Invalidate X and Y velocity if applying input. Used to make maneuvering easier. { /* F2D_Velocity.X = 0.f; F2D_Velocity.Y = 0.f; */ //F2D_Velocity = F2D_InputVector * F2D_VelocityMagnitude; // set velocity in the direction of input F2D_Velocity = F2D_Velocity * SpinJumpHorizontalVelocity; } //F2D_Velocity = F2D_Velocity * 0.65; // dampen velocity Parent->LaunchCharacter({ F2D_Velocity.X, F2D_Velocity.Y, SpinJumpVerticalVelocity }, true, true); break; } case CROUCH_Pressed: NewState(EMoveState::PoundFlipping); return; default: break; } if (CommonJumpActions(input)) { return; } // if (_SpinJumpGravityScaling(input)) { return; } switch (input) { case EVENT_Landed: NewState(EMoveState::Idle); break; case END: GravityScalePeak = __DefaultGravityScalePeak; break; default: break; } } bool UPlayerMovementController::_SpinJumpGravityScaling(EStateInput input) { switch (input) { case TICK: { FVector CapsuleVelocity = Parent->GetCapsuleComponent()->GetComponentVelocity(); if ((CapsuleVelocity.Z < JumpVelocityUpThreshold) && (CapsuleVelocity.Z > JumpVelocityDownTreshold)) { Ref_CharacterMovement->GravityScale = GravityScalePeak / 2.f; } break; } default: break; } return false; } void UPlayerMovementController::Standard_Fall_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (CommonJumpActions(input)) { return; } if (_CoyoteTime(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Falling); break; case TICK: if (!Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Idle); return; } break; case END: break; default: break; } } bool UPlayerMovementController::_CoyoteTime(EStateInput input) { switch (input) { case TICK: _CoyoteTimeAccumulation += _Internal_DeltaTime; break; case JUMP_Pressed: if (_CoyoteTimeAccumulation < CoyoteTimeDuration) { Parent->JumpMaxCount = 2; NewState(EMoveState::Jumping); return true; } break; case END: _CoyoteTimeAccumulation = 0.f; break; default: break; } return false; } void UPlayerMovementController::Standard_Crouch_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crouching); Parent->Crouch(); break; case TICK: if (NormalMovement()) { NewState(EMoveState::Crawling); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Idle); return; case JUMP_Pressed: Parent->UnCrouch(); NewState(EMoveState::HighJumping); return; case END: //Parent->UnCrouch(); //todo: what if transitioning to crawl? does uncrouch, then crouch work? break; case RESET: Parent->UnCrouch(); break; default: break; } } void UPlayerMovementController::Standard_Crawl_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crawling); Parent->Crouch(); break; case TICK: if (!NormalMovement()) { NewState(EMoveState::Crouching); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Running); break; case JUMP_Pressed: Parent->UnCrouch(); NewState(EMoveState::Dodging); return; case END: //Parent->UnCrouch(); break; case RESET: Parent->UnCrouch(); break; default: break; } } void UPlayerMovementController::Standard_Pound_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Pounding); Parent->LaunchCharacter({ 0.f, 0.f, ((DownwardLaunchVelocity <= 0.f) ? DownwardLaunchVelocity : (DownwardLaunchVelocity * -1.f)) }, false, true); break; case EVENT_Landed: NewState(EMoveState::Idle); break; default: break; } } void UPlayerMovementController::Standard_PoundFlip_Implementation(EStateInput input) { switch (input) { case INIT: Parent->_SetMoveState(EMoveState::PoundFlipping); Ref_CharacterMovement->Velocity = { 0.f, 0.f, 0.f }; Ref_CharacterMovement->GravityScale = 0.f; Parent->GetWorldTimerManager().SetTimer(PoundFlipTimer, this, &UPlayerMovementController::PoundFlipTimerHandler, PoundFlipDuration, false); break; case JUMP_Pressed: if (DivesAttempted < MaxDives) { NewState(EMoveState::Diving); } break; case TIMER_PoundFlip: NewState(EMoveState::Pounding); break; case END: Parent->GetWorldTimerManager().ClearTimer(PoundFlipTimer); Ref_CharacterMovement->GravityScale = 1.f; break; default: break; } } void UPlayerMovementController::PoundFlipTimerHandler() { (this->*StateFunction)(EStateInput::TIMER_PoundFlip); } void UPlayerMovementController::Standard_Dive_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (__JumpBuffer(input)) { return; } if (__JumpGravityScaling(input)) { return; } if (__StickyLanding(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Diving); DivesAttempted++; if (Parent->IsApplyingInput()) { // If applying input, dive in input direction FRotator ControlRotation = Parent->GetControlRotation(); FRotator NormalizedControlRotation{ 0.f, ControlRotation.Yaw, 0.f }; FVector ControlRightVector = UKismetMathLibrary::GetRightVector(NormalizedControlRotation); FVector ControlForwardVector = UKismetMathLibrary::GetForwardVector(NormalizedControlRotation); float HorizontalAxisInput = GetHorizontalAxisValue(); float VerticalAxisInput = GetVerticalAxisValue(); FVector InputVector = ((ControlRightVector * HorizontalAxisInput) + (ControlForwardVector * VerticalAxisInput)); // Calc input vector FVector2D Normalized2DInputVector{ InputVector.X, InputVector.Y }; // We want to normalize the 2D components of the input vector UKismetMathLibrary::Normalize2D(Normalized2DInputVector); FVector DiveVector{ (Normalized2DInputVector.X * DiveForwardVelocity), (Normalized2DInputVector.Y * DiveForwardVelocity), DiveZVelocity }; Parent->LaunchCharacter(DiveVector, true, true); } else { // Otherwise, dive in the direction currently facing FVector CapsuleForwardVector = Parent->GetCapsuleComponent()->GetForwardVector(); FVector DiveVector = CapsuleForwardVector * DiveForwardVelocity; DiveVector.Z = DiveZVelocity; Parent->LaunchCharacter(DiveVector, true, true); } break; case CROUCH_Pressed: NewState(EMoveState::PoundFlipping); return; case HIT_Pressed: if (SpinJumpsAttempted < MaxSpinJumps) { Parent->FireHitMechanic(); } break; case EVENT_HitMechanic: NewState(EMoveState::SpinJumping); return; case EVENT_Landed: NewState(EMoveState::Idle); return; default: break; } } void UPlayerMovementController::Standard_Dodge_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (_JumpTransitionGuard(input)) { return; } if (__JumpBuffer(input)) { return; } switch (input) { case INIT: { Parent->_SetMoveState(EMoveState::Dodging); UCapsuleComponent* _CapsuleComponent = Parent->GetCapsuleComponent(); FVector CapsuleForwardVector = _CapsuleComponent->GetForwardVector(); CapsuleForwardVector = CapsuleForwardVector * DodgeForwardVelocity; FVector _LaunchVector{ CapsuleForwardVector.X, CapsuleForwardVector.Y, DodgeUpVelocity }; Parent->LaunchCharacter(_LaunchVector, true, false); break; } case TICK: if (!Ref_CharacterMovement->IsFalling() && !JumpInputBuffered) { NewState(EMoveState::Idle); return; } break; case EVENT_Landed: NewState(EMoveState::Idle); return; default: break; } } bool UPlayerMovementController::CommonActions_Implementation(EStateInput input) { switch(input) { case INIT: break; case HIT_Pressed: Parent->FireHitMechanic(); break; case FLASH_Pressed: Parent->FireCameraMechanic(); break; default: break; } return false; } bool UPlayerMovementController::CommonJumpActions_Implementation(EStateInput input) { if (__JumpBuffer(input)) { return true; } if (__JumpGravityScaling(input)) { return true; } if (__StickyLanding(input)) { return true; } switch (input) { case TICK: AirMovement(); break; case END: //Parent->JumpMaxCount = 1; //Parent->StopJumping(); break; case HIT_Pressed: if (SpinJumpsAttempted < MaxSpinJumps) { Parent->FireHitMechanic(); } break; case FLASH_Pressed: Parent->FireCameraMechanic(); break; case CROUCH_Pressed: NewState(EMoveState::PoundFlipping); return true; case EVENT_HitMechanic: NewState(EMoveState::SpinJumping); return true; default: break; } return false; } bool UPlayerMovementController::__StickyLanding(EStateInput input) { switch (input) { case EVENT_Landed: if (!Parent->IsApplyingInput()) { FVector CharacterMovementVelocity = Ref_CharacterMovement->Velocity; Ref_CharacterMovement->Velocity = FVector(0.f, 0.f, CharacterMovementVelocity.Z); } break; default: break; } return false; } bool UPlayerMovementController::__JumpBuffer(EStateInput input) { switch (input) { case INIT: break; case TICK: if (_JumpBuffered) { _JumpBufferTime += _Internal_DeltaTime; } break; case JUMP_Pressed: if (_JumpBuffered) { _JumpBufferTime = 0.f; } else { _JumpBuffered = true; } break; case EVENT_Landed: if (_JumpBuffered && (_JumpBufferTime < JumpBufferMaxTime)) { NewState(EMoveState::Jumping); return true; } break; case END: _JumpBuffered = false; _JumpBufferTime = 0.f; Parent->StopJumping(); break; default: break; } return false; } bool UPlayerMovementController::__JumpGravityScaling(EStateInput input) { switch (input) { case INIT: break; case TICK: { FVector CapsuleVelocity = Parent->GetCapsuleComponent()->GetComponentVelocity(); if (CapsuleVelocity.Z > JumpVelocityUpThreshold) { Ref_CharacterMovement->GravityScale = GravityScaleUp; } else if (CapsuleVelocity.Z < JumpVelocityDownTreshold) { Ref_CharacterMovement->GravityScale = GravityScaleDown; } else { Ref_CharacterMovement->GravityScale = GravityScalePeak; } break; } case END: Ref_CharacterMovement->GravityScale = 1.f; break; default: break; } return false; } bool UPlayerMovementController::_JumpTransitionGuard(EStateInput input) { switch(input) { case INIT: JumpInputBuffered = true; break; case EVENT_Jumped: //JumpInputBuffered = false; break; case EVENT_Launched: JumpInputBuffered = false; break; case EVENT_Landed: JumpInputBuffered = false; break; case END: JumpInputBuffered = false; break; default: break; } return false; } bool UPlayerMovementController::NormalMovement() { if (Parent->IsApplyingInput()) // todo: does this applying input check have to happen? { FRotator PlayerForwardRotation = UKismetMathLibrary::MakeRotFromX(Parent->GetCapsuleComponent()->GetForwardVector()); /* Rotation between input vector and character forward vector. */ float AbsRotation = _GetAbsoluteRotationBetweenYaws(PlayerForwardRotation, InputRotationFromX); if (Parent->GetVelocityVectorLength() < 300.f) // if moving slowly { _RotateInstantToInputDirection(); // just rotate to the input direction _AddForwardMovement(); } else if (AbsRotation > 170.f) // otherwise, if applying input directly opposite the vector of movement, rotate instantly and skid turn { // todo: skid turn } else // otherwise, rotate slowly and move as normal { _RotateConstantToInputDirection(RotationSpeedSlow); _AddForwardMovement(); } return true; } else { return false; } } bool UPlayerMovementController::AirMovement() { if (Parent->IsApplyingInput()) { _AddRelativeMovement(); _RotateConstantToInputDirection(RotationSpeedSlow); //todo: does extra velocity need to be added? return true; } else { return false; } } void UPlayerMovementController::_AddForwardMovement() { FVector PlayerForwardVector = Parent->GetCapsuleComponent()->GetForwardVector(); FVector RawInputUnitVector; float RawInputVectorLength; Parent->GetRawInputVector().ToDirectionAndLength(OUT RawInputUnitVector, OUT RawInputVectorLength); Parent->AddMovementInput(PlayerForwardVector, RawInputVectorLength, false); } void UPlayerMovementController::_AddRelativeMovement() { FRotator PlayerControlRotation = Parent->GetControlRotation(); FRotator NormalizedControlRotation{ 0.0, PlayerControlRotation.Yaw , 0.0f }; float HorizontalMovement = GetHorizontalAxisValue(); float VerticalMovement = GetVerticalAxisValue(); Parent->AddMovementInput(UKismetMathLibrary::GetRightVector(NormalizedControlRotation), HorizontalMovement, false); Parent->AddMovementInput(UKismetMathLibrary::GetForwardVector(NormalizedControlRotation), VerticalMovement, false); } void UPlayerMovementController::_RotateConstantToInputDirection(float TurnSpeed) { FRotator NewRotationTarget = UKismetMathLibrary::RInterpTo_Constant(Parent->GetCapsuleComponent()->GetComponentRotation(), InputRotationFromX, _Internal_DeltaTime, TurnSpeed); Parent->GetCapsuleComponent()->SetWorldRotation(NewRotationTarget); //UE_LOG(LogTemp, Warning, TEXT("Turning... %f"), _Internal_DeltaTime); } void UPlayerMovementController::_RotateInstantToInputDirection() { Parent->GetCapsuleComponent()->SetWorldRotation(InputRotationFromX); } float UPlayerMovementController::_GetAbsoluteRotationBetweenYaws(FRotator RotA, FRotator RotB) { float RawRotation = FMath::Abs(RotA.Yaw - RotB.Yaw); if (RawRotation > 180.f) { return FMath::Abs(RawRotation - 360.f); } else { return RawRotation; } } void UPlayerMovementController::_SetInputVectorAndRotation() // DEPRECATE { FRotator PlayerControlRotation = Parent->GetControlRotation(); FRotator NormalizedControlRotation{ 0.0f, PlayerControlRotation.Yaw, 0.0f }; float HorizontalMovement = GetHorizontalAxisValue(); float VerticalMovement = GetVerticalAxisValue(); FVector RawInput = (UKismetMathLibrary::GetRightVector(NormalizedControlRotation) * HorizontalMovement) + (UKismetMathLibrary::GetForwardVector(NormalizedControlRotation) * VerticalMovement); RawInputVector = RawInput; RawInput.Normalize(); InputRotationFromX = UKismetMathLibrary::MakeRotFromX(RawInput); } void UPlayerMovementController::_CalculateInputRotationFromX() { InputRotationFromX = UKismetMathLibrary::MakeRotFromX(Parent->GetRawInputVector()); } FVector UPlayerMovementController::GetRawInputVector() { return RawInputVector; } FRotator UPlayerMovementController::GetInputRotationFromX() { return InputRotationFromX; } void UPlayerMovementController::NewState(EMoveState NewMoveState) { (this->*StateFunction)(EStateInput::END); //Parent->_SetMoveState(NewMoveState); //SetMoveState(NewMoveState); switch (NewMoveState) // Update with new states { case Idle: StateFunction = &UPlayerMovementController::State_Idle; break; case Running: StateFunction = &UPlayerMovementController::State_Run; break; case Jumping: StateFunction = &UPlayerMovementController::State_Jump; break; case HighJumping: StateFunction = &UPlayerMovementController::State_HighJump; break; case SpinJumping: StateFunction = &UPlayerMovementController::State_SpinJump; break; case Falling: StateFunction = &UPlayerMovementController::State_Fall; break; case Crouching: StateFunction = &UPlayerMovementController::State_Crouch; break; case Crawling: StateFunction = &UPlayerMovementController::State_Crawl; break; case Pounding: StateFunction = &UPlayerMovementController::State_Pound; break; case PoundFlipping: StateFunction = &UPlayerMovementController::State_PoundFlip; break; case Diving: StateFunction = &UPlayerMovementController::State_Dive; break; case Dodging: StateFunction = &UPlayerMovementController::State_Dodge; break; default: break; } (this->*StateFunction)(EStateInput::INIT); //_Debug_PrintState(); //(this->*StateFunction)(EStateInput::TICK); // todo: should this be called here? } void UPlayerMovementController::NotifyStateChanged_Implementation(EMoveState NewState) { // ... } void UPlayerMovementController::NotifySetChanged_Implementation(EMoveSet NewSet) { NewMoveSet_Notified(NewSet); } void UPlayerMovementController::NotifyOnLanded_Implementation() { (this->*StateFunction)(EStateInput::EVENT_Landed); //Parent->JumpMaxCount = 1; } void UPlayerMovementController::NotifyOnJumped_Implementation() { (this->*StateFunction)(EStateInput::EVENT_Jumped); } void UPlayerMovementController::NotifyOnLaunched_Implementation() { (this->*StateFunction)(EStateInput::EVENT_Launched); } void UPlayerMovementController::NotifyHitMechanicFired_Implementation() { (this->*StateFunction)(EStateInput::EVENT_HitMechanic); } void UPlayerMovementController::NotifyCameraMechanicFired_Implementation() { (this->*StateFunction)(EStateInput::EVENT_CameraMechanic); } void UPlayerMovementController::NewState(void (UPlayerMovementController::* NewStateFunction)(EStateInput e)) { (this->*StateFunction)(EStateInput::END); StateFunction = NewStateFunction; (this->*StateFunction)(EStateInput::INIT); } void UPlayerMovementController::CallStateFunction(EStateInput Input) { (this->*StateFunction)(Input); } void UPlayerMovementController::_Debug_PrintState() { switch (Parent->GetMoveState()) { case Idle: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Idle")); break; case Walking: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Walking")); break; case Running: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Running")); break; case Jumping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Jumping")); break; case HighJumping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: HighJumping")); break; case SpinJumping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: SpinJumping")); break; case Falling: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Falling")); break; case Crouching: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Crouching")); break; case Crawling: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Crawling")); break; case Pounding: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Pounding")); break; case PoundFlipping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: PoundFlipping")); break; case Diving: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Diving")); break; case Dodging: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Dodging")); break; default: break; } } void UPlayerMovementController::NewMoveSet(EMoveSet NewMoveSet) { Parent->_SetMoveSet(NewMoveSet); NewMoveSet_Notified(NewMoveSet); } void UPlayerMovementController::NewMoveSet_Notified(EMoveSet NewMoveSet) { (this->*StateFunction)(EStateInput::SET_END); this->MoveSet = NewMoveSet; (this->*StateFunction)(EStateInput::SET_INIT); } void UPlayerMovementController::Limited_Idle_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Idle); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (NormalMovement()) // If movement is being applied { NewState(EMoveState::Running); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crouching); return; case HIT_Pressed: // fire companion hit (prob in parent APlayer_Character) break; case END: break; default: break; } } void UPlayerMovementController::Limited_Run_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Running); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (!NormalMovement()) { NewState(EMoveState::Idle); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crawling); break; default: break; } } void UPlayerMovementController::Limited_Jump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (_Common_Limited_Jump_Actions(input)) { return; } //if (_RegularJump(input)) { return; } if (_JumpTransitionGuard(input)) { return; } switch (input) { case SET_INIT: Ref_CharacterMovement->JumpZVelocity = 300.f; break; case INIT: StandardJumpZVelocity = Ref_CharacterMovement->JumpZVelocity; Ref_CharacterMovement->JumpZVelocity = 300.f; Parent->Jump(); Parent->_SetMoveState(EMoveState::Jumping); if (!Parent->CanJump()) { NewState(EMoveState::Idle); return; } break; case TICK: if (!Ref_CharacterMovement->IsFalling() && (!JumpInputBuffered || !Parent->CanJump())) //todo: can you refactor this to EVENT_Landed? { NewState(EMoveState::Idle); return; } break; case JUMP_Released: Parent->StopJumping(); break; case EVENT_Landed: Parent->StopJumping(); break; case END: case SET_END: Ref_CharacterMovement->JumpZVelocity = StandardJumpZVelocity; break; default: break; } } bool UPlayerMovementController::_Common_Limited_Jump_Actions(EStateInput input) { if (__JumpBuffer(input)) { return true; } if (__JumpGravityScaling(input)) { return true; } if (__StickyLanding(input)) { return true; } switch (input) { case TICK: AirMovement(); break; case END: //Parent->JumpMaxCount = 1; //Parent->StopJumping(); break; case FLASH_Pressed: Parent->FireCameraMechanic(); break; default: break; } return false; } void UPlayerMovementController::Limited_Fall_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (_Common_Limited_Jump_Actions(input)) { return; } if (_CoyoteTime(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Falling); break; case TICK: if (!Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Idle); return; } break; case END: break; default: break; } } void UPlayerMovementController::Limited_Crouch_Implementation(EStateInput input) { if (_CommonActions_Limited(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crouching); Parent->Crouch(); break; case TICK: if (NormalMovement()) { NewState(EMoveState::Crawling); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Idle); return; case END: //Parent->UnCrouch(); //todo: what if transitioning to crawl? does uncrouch, then crouch work? break; case RESET: Parent->UnCrouch(); break; default: break; } } bool UPlayerMovementController::_CommonActions_Limited(EStateInput input) { switch (input) { case FLASH_Pressed: Parent->FireCameraMechanic(); break; default: break; } return false; } void UPlayerMovementController::Limited_Crawl_Implementation(EStateInput input) { if (_CommonActions_Limited(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crawling); Parent->Crouch(); break; case TICK: if (!NormalMovement()) { NewState(EMoveState::Crouching); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Running); break; case END: //Parent->UnCrouch(); break; case RESET: Parent->UnCrouch(); break; default: break; } }
Iterative Playtesting
Methodology
The only way to “find the fun” and know that a game mechanic or level idea is successful is to put it in front of players and test it.
I implemented an iterative testing cycle alongside our user experience team to capture player feedback continuously throughout development, iterating as we went.
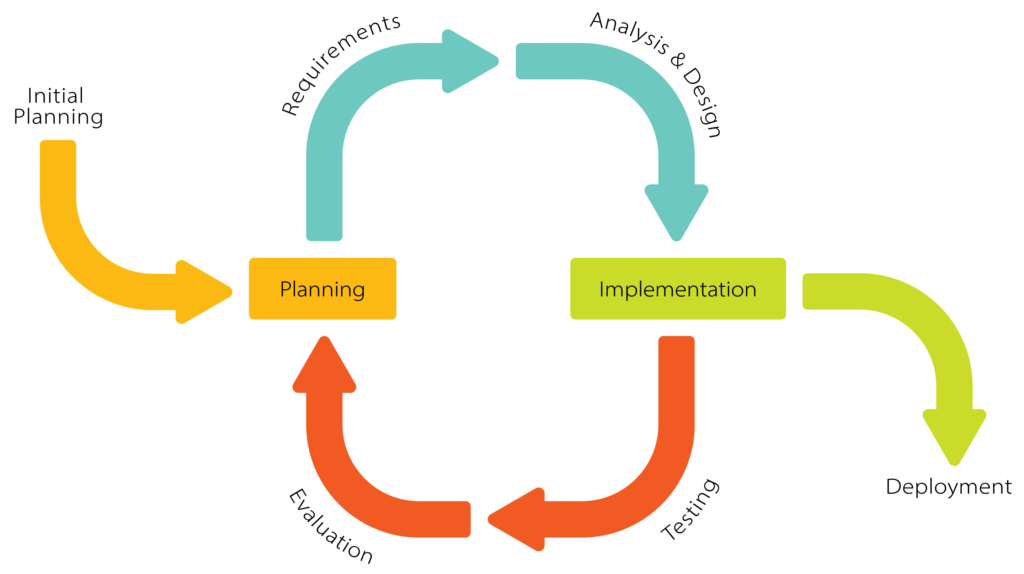
Playtest Process
Playtest feedback is aggregated on sticky notes using Miro. This lets us organize the feedback we have received and take notes, showing us at a glance where trouble spots or problem mechanics are.
From here, we can build a priority queue of changes to improve on for the next iteration.
All feedback from each iteration is pooled together on one virtual board, so we can see how the mechanics and levels are improving from week to week, and specifically note regressions or observe trends.
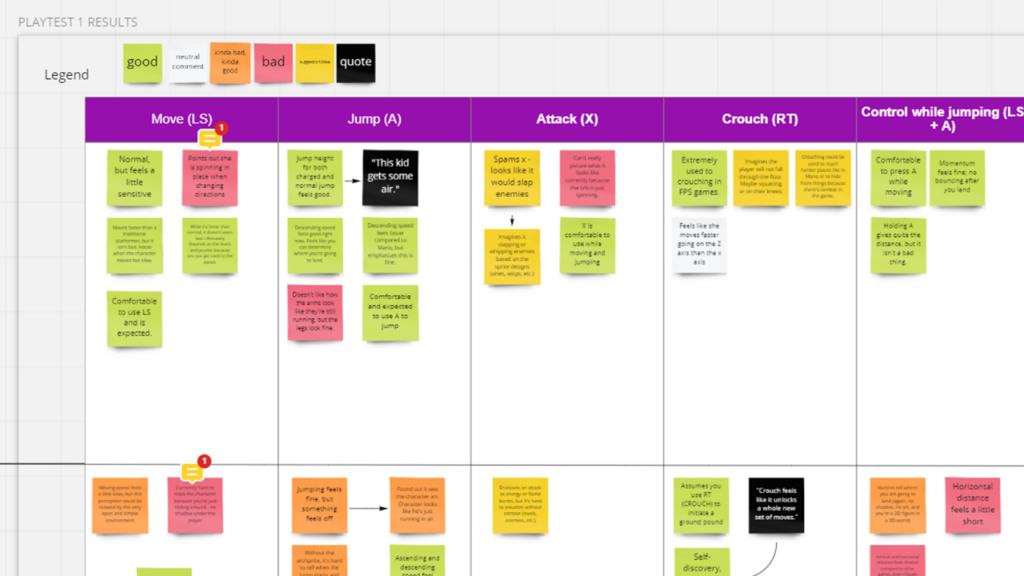
Blueprint/C++ Integration
A Combined Approach
C++ and Blueprints work together to enable fast iteration and high performance. Our early prototypes began as Blueprint code and were later refactored to C++ to optimize for performance.
Integrating C++ and Blueprints
All gameplay objects are children of base C++ classes.
Unreal C++ macros are used to demarcate functions and variables that should be exposed to Blueprint code in the child classes.
This allows us to write custom Blueprint nodes in C++, and then use them in the Blueprint node graph to quickly create prototypes or new functionality.
Ensuring gameplay objects are Blueprint children also enables us to utilize Unreal features that are faster in Blueprint, such as editing an object”s components or using asynchronous processes such as Timelines.
A combined approach saves time and effort for programmers and designers, while maintaining high performance.
Character Finite State Machine
Performance and Extensibility
A finite state system provides optimized performance for the player input system. Using discrete states and function pointers, we can isolate functionality and extend it as needed while saving conditional checks.
Representing States via Enums
The enumerator EMoveState defines the discrete movement states the player may be in at any given time.
Then, the actual behavior is defined by a MoveSet. The enum EMoveSet defines the actual movesets, and can be expanded to as needed. These movesets point the function pointers to the state functions for the given MoveSet.
In this example, we have a Standard moveset that the player typically uses. At certain times, we want to limit a player’s actions. For that, we use the “Limited” moveset and define in code a different set of state functions for what “Limited” movement looks like.
Handling Input
All player and engine input is routed through a custom input handling function. The enum EStateInput defines each input, and this input is passed to each state function. The state function then handles behavior or transitions based on the input given.
Inputs include buttons the player presses, as well as engine events such as the event tick.
UENUM(BlueprintType)
enum EMoveState
{
Idle UMETA(DisplayName = "Idle"),
Walking UMETA(DisplayName = "Walking"),
Running UMETA(DisplayName = "Running"),
Jumping UMETA(DisplayName = "Jumping"),
HighJumping UMETA(DisplayName = "HighJumping"),
SpinJumping UMETA(DisplayName = "SpinJumping"),
Falling UMETA(DisplayName = "Falling"),
Crouching UMETA(DisplayName = "Crouching"),
Crawling UMETA(DisplayName = "Crawling"),
Pounding UMETA(DisplayName = "Pounding"),
PoundFlipping UMETA(DisplayName = "PoundFlipping"),
Diving UMETA(DisplayName = "Diving"),
Dodging UMETA(DisplayName = "Dodging"),
};
UENUM(BlueprintType)
enum EMoveSet
{
Standard UMETA(DisplayName = "Standard"),
Limited UMETA(DisplayName = "Limited"),
};
/**
* This represents all possible inputs for each
* movement state that the player can be in.
*/
UENUM(BlueprintType)
enum EStateInput
{
TICK,
INIT,
END,
SET_INIT,
SET_END,
JUMP_Pressed,
JUMP_Released,
CROUCH_Pressed,
CROUCH_Released,
FLASH_Pressed,
FLASH_Released,
GRAB_Pressed,
GRAB_Released,
HIT_Pressed,
HIT_Released,
AXIS,
AXIS_HORIZONTAL,
AXIS_VERTICAL,
EVENT_Landed,
EVENT_Jumped,
EVENT_Launched,
EVENT_HitMechanic,
EVENT_CameraMechanic,
TIMER_PoundFlip,
RESET,
};
Minimize Clock Cycles with Delegates and Function Pointers
Input and gameplay logic is handled in an event-based manner. This minimizes the need for costly functions to run in the frame tick.
Player movement logic is kept in atomic handler functions, and when the player’s moveset changes, a function delegate is given a pointer to the next handler function. That function then receives player input and engine frame ticks, preventing the need for extraneous if statements or state checks.
// Jump input is abstracted and passed as a state transition into the state machine.
void UPlayerMovementController::JumpActionPressed()
{
if (!InputEnabled) { return; }
(this->*StateFunction)(EStateInput::JUMP_Pressed);
}
// Designed to be extended with new movesets.
void UPlayerMovementController::State_Jump_Implementation(EStateInput input)
{
switch (MoveSet)
{
case Limited:
Limited_Jump(input);
break;
default:
Standard_Jump(input);
break;
}
}
// Copyright 2022 Soup Initiative Games #include "ProjectSpooky64/Public/Pawns/PlayerMovementController.h" #include "ProjectSpooky64/Public/Pawns/APlayer_Character.h" #include "PaperCharacter.h" #include "Components/CapsuleComponent.h" #include "GameFramework/Actor.h" #include "Kismet/KismetMathLibrary.h" #include "Kismet/GameplayStatics.h" // Sets default values for this component's properties UPlayerMovementController::UPlayerMovementController() { // Set this component to be initialized when the game starts, and to be ticked every frame. You can turn these features // off to improve performance if you don't need them. PrimaryComponentTick.bCanEverTick = true; // ... MoveState = EMoveState::Idle; MoveSet = EMoveSet::Standard; } // Called when the game starts void UPlayerMovementController::BeginPlay() { Super::BeginPlay(); //Ref_CharacterMovement = GetOwner()->FindComponentByClass<UCharacterMovementComponent>(); Parent = Cast<AAPlayer_Character>(GetOwner()); Ref_CharacterMovement = Parent->GetCharacterMovement(); Parent->JumpMaxHoldTime = JumpBufferMaxTime; Parent->OnMoveStateChanged.AddDynamic(this, &UPlayerMovementController::NotifyStateChanged); Parent->OnMoveSetChanged.AddDynamic(this, &UPlayerMovementController::NotifySetChanged); Parent->OnLanded.AddDynamic(this, &UPlayerMovementController::NotifyOnLanded); Parent->OnJumped.AddDynamic(this, &UPlayerMovementController::NotifyOnJumped); Parent->OnLaunched.AddDynamic(this, &UPlayerMovementController::NotifyOnLaunched); Parent->HitMechanic.AddDynamic(this, &UPlayerMovementController::NotifyHitMechanicFired); Parent->CameraMechanic.AddDynamic(this, &UPlayerMovementController::NotifyCameraMechanicFired); StateFunction = &UPlayerMovementController::State_Idle; (this->*StateFunction)(EStateInput::INIT); } // Called every frame void UPlayerMovementController::TickComponent(float DeltaTime, ELevelTick TickType, FActorComponentTickFunction* ThisTickFunction) { Super::TickComponent(DeltaTime, TickType, ThisTickFunction); //UE_LOG(LogTemp, Warning, TEXT("Jump buffered: %s"), (JumpInputBuffered) ? TEXT("TRUE") : TEXT("false")); } void UPlayerMovementController::GuardedTick_Implementation(float DeltaTime) { _Internal_DeltaTime = DeltaTime; //_SetInputVectorAndRotation(); // todo: might be able to move this to a lower function later _CalculateInputRotationFromX(); (this->*StateFunction)(EStateInput::TICK); //UE_LOG(LogTemp, Warning, TEXT("Can the parent jump: %s"), (Parent->CanJump() ? TEXT("true") : TEXT("false"))); //_Debug_PrintState(); } float UPlayerMovementController::GetInternalDeltaTime() { return _Internal_DeltaTime; } void UPlayerMovementController::SetMoveState(EMoveState NewState) { MoveState = NewState; } void UPlayerMovementController::SetMoveSet(EMoveSet NewSet) { MoveSet = NewSet; } EMoveState UPlayerMovementController::GetMoveState() { return MoveState.GetValue(); } EMoveSet UPlayerMovementController::GetMoveSet() { return MoveSet.GetValue(); } void UPlayerMovementController::InitializeInputs(UInputComponent* PlayerInputComponent) { UE_LOG(LogTemp, Warning, TEXT("PlayerMovementController initialized.")); PlayerInputComponent->BindAction("Jump", EInputEvent::IE_Pressed, this, &UPlayerMovementController::JumpActionPressed); PlayerInputComponent->BindAction("Jump", EInputEvent::IE_Released, this, &UPlayerMovementController::JumpActionReleased); PlayerInputComponent->BindAction("Crouch", EInputEvent::IE_Pressed, this, &UPlayerMovementController::CrouchActionPressed); PlayerInputComponent->BindAction("Crouch", EInputEvent::IE_Released, this, &UPlayerMovementController::CrouchActionReleased); PlayerInputComponent->BindAction("Flash", EInputEvent::IE_Pressed, this, &UPlayerMovementController::FlashActionPressed); PlayerInputComponent->BindAction("Flash", EInputEvent::IE_Released, this, &UPlayerMovementController::FlashActionReleased); PlayerInputComponent->BindAction("Grab", EInputEvent::IE_Pressed, this, &UPlayerMovementController::GrabActionPressed); PlayerInputComponent->BindAction("Grab", EInputEvent::IE_Released, this, &UPlayerMovementController::GrabActionReleased); PlayerInputComponent->BindAction("Hit", EInputEvent::IE_Pressed, this, &UPlayerMovementController::HitActionPressed); PlayerInputComponent->BindAction("Hit", EInputEvent::IE_Released, this, &UPlayerMovementController::HitActionReleased); PlayerInputComponent->BindAxis("HorizontalMovement", this, &UPlayerMovementController::HorizontalAxisInput); PlayerInputComponent->BindAxis("VerticalMovement", this, &UPlayerMovementController::VerticalAxisInput); } void UPlayerMovementController::JumpActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::JUMP_Pressed); } void UPlayerMovementController::JumpActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::JUMP_Released); } void UPlayerMovementController::CrouchActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::CROUCH_Pressed); //UE_LOG(LogTemp, Warning, TEXT("Crouch action pressed")); } void UPlayerMovementController::CrouchActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::CROUCH_Released); } void UPlayerMovementController::FlashActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::FLASH_Pressed); } void UPlayerMovementController::FlashActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::FLASH_Released); Parent->ReleaseCameraMechanic(); } void UPlayerMovementController::GrabActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::GRAB_Pressed); Parent->FireAimMechanic(); // Might want to refactor into a state helper function. UE_LOG(LogTemp, Warning, TEXT("Grab action pressed")); } void UPlayerMovementController::GrabActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::GRAB_Released); Parent->ReleaseAimMechanic(); // Might want to refactor into a state helper function. UE_LOG(LogTemp, Warning, TEXT("Grab action released")); } void UPlayerMovementController::HitActionPressed() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::HIT_Pressed); } void UPlayerMovementController::HitActionReleased() { if (!InputEnabled) { return; } (this->*StateFunction)(EStateInput::HIT_Released); Parent->ReleaseHitMechanic(); } void UPlayerMovementController::HorizontalAxisInput(float AxisValue) { } void UPlayerMovementController::VerticalAxisInput(float AxisValue) { } void UPlayerMovementController::SetMoveEnabled(bool Enabled) { if (Enabled) { this->InputEnabled = true; Parent->_SetMovementInputEnabled(true); } else { this->InputEnabled = false; Parent->_SetMovementInputEnabled(false); ResetMovement(); } } float UPlayerMovementController::GetHorizontalAxisValue() { if (!InputEnabled) { return 0.f; } return Parent->GetInputAxisValue("HorizontalMovement"); } float UPlayerMovementController::GetVerticalAxisValue() { if (!InputEnabled) { return 0.f; } return Parent->GetInputAxisValue("VerticalMovement"); } void UPlayerMovementController::ResetMovement() { (this->*StateFunction)(EStateInput::RESET); NewState(EMoveState::Idle); Ref_CharacterMovement->Velocity = FVector{ 0.f, 0.f, 0.f }; } void UPlayerMovementController::State_Idle_Implementation(EStateInput input) { switch(MoveSet) { case Limited: Limited_Idle(input); break; default: Standard_Idle(input); break; } } void UPlayerMovementController::State_Run_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Run(input); break; default: Standard_Run(input); break; } } void UPlayerMovementController::State_Jump_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Jump(input); break; default: Standard_Jump(input); break; } } void UPlayerMovementController::State_HighJump_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_HighJump(input); break; } } void UPlayerMovementController::State_SpinJump_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_SpinJump(input); break; } } void UPlayerMovementController::State_Fall_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Fall(input); break; default: Standard_Fall(input); break; } } void UPlayerMovementController::State_Crouch_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Crouch(input); break; default: Standard_Crouch(input); break; } } void UPlayerMovementController::State_Crawl_Implementation(EStateInput input) { switch (MoveSet) { case Limited: Limited_Crawl(input); break; default: Standard_Crawl(input); break; } } void UPlayerMovementController::State_Pound_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_Pound(input); break; } } void UPlayerMovementController::State_PoundFlip_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_PoundFlip(input); break; } } void UPlayerMovementController::State_Dive_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_Dive(input); break; } } void UPlayerMovementController::State_Dodge_Implementation(EStateInput input) { switch (MoveSet) { case Limited: break; default: Standard_Dodge(input); break; } } /* * Note: * When extending in Blueprint, * call Super/parent version for every case that isn't going to have a unique Blueprint implementation. */ void UPlayerMovementController::Standard_Idle_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Idle); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (NormalMovement()) // If movement is being applied { NewState(EMoveState::Running); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crouching); return; case HIT_Pressed: // fire companion hit (prob in parent APlayer_Character) break; case END: break; default: break; } } void UPlayerMovementController::Standard_Run_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Running); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (!NormalMovement()) { NewState(EMoveState::Idle); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crawling); break; default: break; } } void UPlayerMovementController::Standard_Jump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } /* * Returns true if NewState is called in CommonJumpAction's scope, therefore preventing the remainder of this function * from firing after the state has been changed. */ if (CommonJumpActions(input)) { return; } if (_RegularJump(input)) { return; } if (_JumpTransitionGuard(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Jumping); //UE_LOG(LogTemp, Warning, TEXT("Jump max count: %i"), Parent->JumpMaxCount); //Parent->Jump(); //CharMovement_JumpBuffered = true; // Guard for notifying TICK case that there is a jump waiting to be applied next update if (!Parent->CanJump()) { NewState(EMoveState::Idle); return; } break; case TICK: //UE_LOG(LogTemp, Warning, TEXT("Jump buffered: %s"), (JumpInputBuffered) ? TEXT("TRUE") : TEXT("false")); if (!Ref_CharacterMovement->IsFalling() && (!JumpInputBuffered || !Parent->CanJump())) //todo: can you refactor this to EVENT_Landed? { NewState(EMoveState::Idle); return; } /*todo: if jump is called but cannot be processed, call idle*/ //CharMovement_JumpBuffered = false; break; case JUMP_Released: Parent->StopJumping(); break; case CROUCH_Released: break; case EVENT_Landed: Parent->StopJumping(); break; case EVENT_Jumped: //CharMovement_JumpBuffered = false; break; case END: //CharMovement_JumpBuffered = true; //Parent->JumpMaxCount = 1; break; default: break; } } bool UPlayerMovementController::_RegularJump(EStateInput input) { switch (input) { case INIT: Parent->Jump(); break; case END: Parent->JumpMaxCount = 1; break; default: break; } return false; } bool UPlayerMovementController::CommonOnLandedActions_Implementation(EStateInput input) { switch (input) { case RESET: case EVENT_Landed: SpinJumpsAttempted = 0; DivesAttempted = 0; Parent->StopJumping(); break; default: break; } return false; } void UPlayerMovementController::Standard_HighJump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (CommonJumpActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::HighJumping); Parent->LaunchCharacter(FVector(0.f, 0.f, Ref_CharacterMovement->JumpZVelocity * 1.75), true, true); break; case TICK: break; case EVENT_Landed: //todo: is this going to cause errors? revisit if errors occur around highjumping NewState(EMoveState::Idle); return; default: break; } } void UPlayerMovementController::Standard_SpinJump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } switch (input) { case INIT: { SpinJumpsAttempted++; Parent->_SetMoveState(EMoveState::SpinJumping); __DefaultGravityScalePeak = GravityScalePeak; // Note: Alters state variables here, which are reset once this state ends. GravityScalePeak = GravityScalePeak / 2.f; FVector _Velocity = Ref_CharacterMovement->Velocity; FVector2D F2D_Velocity{ _Velocity.X, _Velocity.Y }; /* FVector InputVector = InputRotationFromX.Vector(); // get input from player forward vector FVector2D F2D_InputVector{ InputVector.X, InputVector.Y }; // make 2D F2D_Velocity = F2D_Velocity * F2D_InputVector; // @todo: this should redirect velocity in direction of movement */ FVector2D F2D_VelocityDirection{ 0.f }; float F2D_VelocityMagnitude = 0.f; // we want the magnitude F2D_Velocity.ToDirectionAndLength(F2D_VelocityDirection, F2D_VelocityMagnitude); FVector2D F2D_InputVector{ RawInputVector.X, RawInputVector.Y }; // get raw input vector 2D FVector InputVect = InputRotationFromX.Vector(); F2D_Velocity.X = InputVect.X; F2D_Velocity.Y = InputVect.Y; if (Parent->IsApplyingInput()) // Invalidate X and Y velocity if applying input. Used to make maneuvering easier. { /* F2D_Velocity.X = 0.f; F2D_Velocity.Y = 0.f; */ //F2D_Velocity = F2D_InputVector * F2D_VelocityMagnitude; // set velocity in the direction of input F2D_Velocity = F2D_Velocity * SpinJumpHorizontalVelocity; } //F2D_Velocity = F2D_Velocity * 0.65; // dampen velocity Parent->LaunchCharacter({ F2D_Velocity.X, F2D_Velocity.Y, SpinJumpVerticalVelocity }, true, true); break; } case CROUCH_Pressed: NewState(EMoveState::PoundFlipping); return; default: break; } if (CommonJumpActions(input)) { return; } // if (_SpinJumpGravityScaling(input)) { return; } switch (input) { case EVENT_Landed: NewState(EMoveState::Idle); break; case END: GravityScalePeak = __DefaultGravityScalePeak; break; default: break; } } bool UPlayerMovementController::_SpinJumpGravityScaling(EStateInput input) { switch (input) { case TICK: { FVector CapsuleVelocity = Parent->GetCapsuleComponent()->GetComponentVelocity(); if ((CapsuleVelocity.Z < JumpVelocityUpThreshold) && (CapsuleVelocity.Z > JumpVelocityDownTreshold)) { Ref_CharacterMovement->GravityScale = GravityScalePeak / 2.f; } break; } default: break; } return false; } void UPlayerMovementController::Standard_Fall_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (CommonJumpActions(input)) { return; } if (_CoyoteTime(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Falling); break; case TICK: if (!Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Idle); return; } break; case END: break; default: break; } } bool UPlayerMovementController::_CoyoteTime(EStateInput input) { switch (input) { case TICK: _CoyoteTimeAccumulation += _Internal_DeltaTime; break; case JUMP_Pressed: if (_CoyoteTimeAccumulation < CoyoteTimeDuration) { Parent->JumpMaxCount = 2; NewState(EMoveState::Jumping); return true; } break; case END: _CoyoteTimeAccumulation = 0.f; break; default: break; } return false; } void UPlayerMovementController::Standard_Crouch_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crouching); Parent->Crouch(); break; case TICK: if (NormalMovement()) { NewState(EMoveState::Crawling); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Idle); return; case JUMP_Pressed: Parent->UnCrouch(); NewState(EMoveState::HighJumping); return; case END: //Parent->UnCrouch(); //todo: what if transitioning to crawl? does uncrouch, then crouch work? break; case RESET: Parent->UnCrouch(); break; default: break; } } void UPlayerMovementController::Standard_Crawl_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crawling); Parent->Crouch(); break; case TICK: if (!NormalMovement()) { NewState(EMoveState::Crouching); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Running); break; case JUMP_Pressed: Parent->UnCrouch(); NewState(EMoveState::Dodging); return; case END: //Parent->UnCrouch(); break; case RESET: Parent->UnCrouch(); break; default: break; } } void UPlayerMovementController::Standard_Pound_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Pounding); Parent->LaunchCharacter({ 0.f, 0.f, ((DownwardLaunchVelocity <= 0.f) ? DownwardLaunchVelocity : (DownwardLaunchVelocity * -1.f)) }, false, true); break; case EVENT_Landed: NewState(EMoveState::Idle); break; default: break; } } void UPlayerMovementController::Standard_PoundFlip_Implementation(EStateInput input) { switch (input) { case INIT: Parent->_SetMoveState(EMoveState::PoundFlipping); Ref_CharacterMovement->Velocity = { 0.f, 0.f, 0.f }; Ref_CharacterMovement->GravityScale = 0.f; Parent->GetWorldTimerManager().SetTimer(PoundFlipTimer, this, &UPlayerMovementController::PoundFlipTimerHandler, PoundFlipDuration, false); break; case JUMP_Pressed: if (DivesAttempted < MaxDives) { NewState(EMoveState::Diving); } break; case TIMER_PoundFlip: NewState(EMoveState::Pounding); break; case END: Parent->GetWorldTimerManager().ClearTimer(PoundFlipTimer); Ref_CharacterMovement->GravityScale = 1.f; break; default: break; } } void UPlayerMovementController::PoundFlipTimerHandler() { (this->*StateFunction)(EStateInput::TIMER_PoundFlip); } void UPlayerMovementController::Standard_Dive_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (__JumpBuffer(input)) { return; } if (__JumpGravityScaling(input)) { return; } if (__StickyLanding(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Diving); DivesAttempted++; if (Parent->IsApplyingInput()) { // If applying input, dive in input direction FRotator ControlRotation = Parent->GetControlRotation(); FRotator NormalizedControlRotation{ 0.f, ControlRotation.Yaw, 0.f }; FVector ControlRightVector = UKismetMathLibrary::GetRightVector(NormalizedControlRotation); FVector ControlForwardVector = UKismetMathLibrary::GetForwardVector(NormalizedControlRotation); float HorizontalAxisInput = GetHorizontalAxisValue(); float VerticalAxisInput = GetVerticalAxisValue(); FVector InputVector = ((ControlRightVector * HorizontalAxisInput) + (ControlForwardVector * VerticalAxisInput)); // Calc input vector FVector2D Normalized2DInputVector{ InputVector.X, InputVector.Y }; // We want to normalize the 2D components of the input vector UKismetMathLibrary::Normalize2D(Normalized2DInputVector); FVector DiveVector{ (Normalized2DInputVector.X * DiveForwardVelocity), (Normalized2DInputVector.Y * DiveForwardVelocity), DiveZVelocity }; Parent->LaunchCharacter(DiveVector, true, true); } else { // Otherwise, dive in the direction currently facing FVector CapsuleForwardVector = Parent->GetCapsuleComponent()->GetForwardVector(); FVector DiveVector = CapsuleForwardVector * DiveForwardVelocity; DiveVector.Z = DiveZVelocity; Parent->LaunchCharacter(DiveVector, true, true); } break; case CROUCH_Pressed: NewState(EMoveState::PoundFlipping); return; case HIT_Pressed: if (SpinJumpsAttempted < MaxSpinJumps) { Parent->FireHitMechanic(); } break; case EVENT_HitMechanic: NewState(EMoveState::SpinJumping); return; case EVENT_Landed: NewState(EMoveState::Idle); return; default: break; } } void UPlayerMovementController::Standard_Dodge_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (_JumpTransitionGuard(input)) { return; } if (__JumpBuffer(input)) { return; } switch (input) { case INIT: { Parent->_SetMoveState(EMoveState::Dodging); UCapsuleComponent* _CapsuleComponent = Parent->GetCapsuleComponent(); FVector CapsuleForwardVector = _CapsuleComponent->GetForwardVector(); CapsuleForwardVector = CapsuleForwardVector * DodgeForwardVelocity; FVector _LaunchVector{ CapsuleForwardVector.X, CapsuleForwardVector.Y, DodgeUpVelocity }; Parent->LaunchCharacter(_LaunchVector, true, false); break; } case TICK: if (!Ref_CharacterMovement->IsFalling() && !JumpInputBuffered) { NewState(EMoveState::Idle); return; } break; case EVENT_Landed: NewState(EMoveState::Idle); return; default: break; } } bool UPlayerMovementController::CommonActions_Implementation(EStateInput input) { switch(input) { case INIT: break; case HIT_Pressed: Parent->FireHitMechanic(); break; case FLASH_Pressed: Parent->FireCameraMechanic(); break; default: break; } return false; } bool UPlayerMovementController::CommonJumpActions_Implementation(EStateInput input) { if (__JumpBuffer(input)) { return true; } if (__JumpGravityScaling(input)) { return true; } if (__StickyLanding(input)) { return true; } switch (input) { case TICK: AirMovement(); break; case END: //Parent->JumpMaxCount = 1; //Parent->StopJumping(); break; case HIT_Pressed: if (SpinJumpsAttempted < MaxSpinJumps) { Parent->FireHitMechanic(); } break; case FLASH_Pressed: Parent->FireCameraMechanic(); break; case CROUCH_Pressed: NewState(EMoveState::PoundFlipping); return true; case EVENT_HitMechanic: NewState(EMoveState::SpinJumping); return true; default: break; } return false; } bool UPlayerMovementController::__StickyLanding(EStateInput input) { switch (input) { case EVENT_Landed: if (!Parent->IsApplyingInput()) { FVector CharacterMovementVelocity = Ref_CharacterMovement->Velocity; Ref_CharacterMovement->Velocity = FVector(0.f, 0.f, CharacterMovementVelocity.Z); } break; default: break; } return false; } bool UPlayerMovementController::__JumpBuffer(EStateInput input) { switch (input) { case INIT: break; case TICK: if (_JumpBuffered) { _JumpBufferTime += _Internal_DeltaTime; } break; case JUMP_Pressed: if (_JumpBuffered) { _JumpBufferTime = 0.f; } else { _JumpBuffered = true; } break; case EVENT_Landed: if (_JumpBuffered && (_JumpBufferTime < JumpBufferMaxTime)) { NewState(EMoveState::Jumping); return true; } break; case END: _JumpBuffered = false; _JumpBufferTime = 0.f; Parent->StopJumping(); break; default: break; } return false; } bool UPlayerMovementController::__JumpGravityScaling(EStateInput input) { switch (input) { case INIT: break; case TICK: { FVector CapsuleVelocity = Parent->GetCapsuleComponent()->GetComponentVelocity(); if (CapsuleVelocity.Z > JumpVelocityUpThreshold) { Ref_CharacterMovement->GravityScale = GravityScaleUp; } else if (CapsuleVelocity.Z < JumpVelocityDownTreshold) { Ref_CharacterMovement->GravityScale = GravityScaleDown; } else { Ref_CharacterMovement->GravityScale = GravityScalePeak; } break; } case END: Ref_CharacterMovement->GravityScale = 1.f; break; default: break; } return false; } bool UPlayerMovementController::_JumpTransitionGuard(EStateInput input) { switch(input) { case INIT: JumpInputBuffered = true; break; case EVENT_Jumped: //JumpInputBuffered = false; break; case EVENT_Launched: JumpInputBuffered = false; break; case EVENT_Landed: JumpInputBuffered = false; break; case END: JumpInputBuffered = false; break; default: break; } return false; } bool UPlayerMovementController::NormalMovement() { if (Parent->IsApplyingInput()) // todo: does this applying input check have to happen? { FRotator PlayerForwardRotation = UKismetMathLibrary::MakeRotFromX(Parent->GetCapsuleComponent()->GetForwardVector()); /* Rotation between input vector and character forward vector. */ float AbsRotation = _GetAbsoluteRotationBetweenYaws(PlayerForwardRotation, InputRotationFromX); if (Parent->GetVelocityVectorLength() < 300.f) // if moving slowly { _RotateInstantToInputDirection(); // just rotate to the input direction _AddForwardMovement(); } else if (AbsRotation > 170.f) // otherwise, if applying input directly opposite the vector of movement, rotate instantly and skid turn { // todo: skid turn } else // otherwise, rotate slowly and move as normal { _RotateConstantToInputDirection(RotationSpeedSlow); _AddForwardMovement(); } return true; } else { return false; } } bool UPlayerMovementController::AirMovement() { if (Parent->IsApplyingInput()) { _AddRelativeMovement(); _RotateConstantToInputDirection(RotationSpeedSlow); //todo: does extra velocity need to be added? return true; } else { return false; } } void UPlayerMovementController::_AddForwardMovement() { FVector PlayerForwardVector = Parent->GetCapsuleComponent()->GetForwardVector(); FVector RawInputUnitVector; float RawInputVectorLength; Parent->GetRawInputVector().ToDirectionAndLength(OUT RawInputUnitVector, OUT RawInputVectorLength); Parent->AddMovementInput(PlayerForwardVector, RawInputVectorLength, false); } void UPlayerMovementController::_AddRelativeMovement() { FRotator PlayerControlRotation = Parent->GetControlRotation(); FRotator NormalizedControlRotation{ 0.0, PlayerControlRotation.Yaw , 0.0f }; float HorizontalMovement = GetHorizontalAxisValue(); float VerticalMovement = GetVerticalAxisValue(); Parent->AddMovementInput(UKismetMathLibrary::GetRightVector(NormalizedControlRotation), HorizontalMovement, false); Parent->AddMovementInput(UKismetMathLibrary::GetForwardVector(NormalizedControlRotation), VerticalMovement, false); } void UPlayerMovementController::_RotateConstantToInputDirection(float TurnSpeed) { FRotator NewRotationTarget = UKismetMathLibrary::RInterpTo_Constant(Parent->GetCapsuleComponent()->GetComponentRotation(), InputRotationFromX, _Internal_DeltaTime, TurnSpeed); Parent->GetCapsuleComponent()->SetWorldRotation(NewRotationTarget); //UE_LOG(LogTemp, Warning, TEXT("Turning... %f"), _Internal_DeltaTime); } void UPlayerMovementController::_RotateInstantToInputDirection() { Parent->GetCapsuleComponent()->SetWorldRotation(InputRotationFromX); } float UPlayerMovementController::_GetAbsoluteRotationBetweenYaws(FRotator RotA, FRotator RotB) { float RawRotation = FMath::Abs(RotA.Yaw - RotB.Yaw); if (RawRotation > 180.f) { return FMath::Abs(RawRotation - 360.f); } else { return RawRotation; } } void UPlayerMovementController::_SetInputVectorAndRotation() // DEPRECATE { FRotator PlayerControlRotation = Parent->GetControlRotation(); FRotator NormalizedControlRotation{ 0.0f, PlayerControlRotation.Yaw, 0.0f }; float HorizontalMovement = GetHorizontalAxisValue(); float VerticalMovement = GetVerticalAxisValue(); FVector RawInput = (UKismetMathLibrary::GetRightVector(NormalizedControlRotation) * HorizontalMovement) + (UKismetMathLibrary::GetForwardVector(NormalizedControlRotation) * VerticalMovement); RawInputVector = RawInput; RawInput.Normalize(); InputRotationFromX = UKismetMathLibrary::MakeRotFromX(RawInput); } void UPlayerMovementController::_CalculateInputRotationFromX() { InputRotationFromX = UKismetMathLibrary::MakeRotFromX(Parent->GetRawInputVector()); } FVector UPlayerMovementController::GetRawInputVector() { return RawInputVector; } FRotator UPlayerMovementController::GetInputRotationFromX() { return InputRotationFromX; } void UPlayerMovementController::NewState(EMoveState NewMoveState) { (this->*StateFunction)(EStateInput::END); //Parent->_SetMoveState(NewMoveState); //SetMoveState(NewMoveState); switch (NewMoveState) // Update with new states { case Idle: StateFunction = &UPlayerMovementController::State_Idle; break; case Running: StateFunction = &UPlayerMovementController::State_Run; break; case Jumping: StateFunction = &UPlayerMovementController::State_Jump; break; case HighJumping: StateFunction = &UPlayerMovementController::State_HighJump; break; case SpinJumping: StateFunction = &UPlayerMovementController::State_SpinJump; break; case Falling: StateFunction = &UPlayerMovementController::State_Fall; break; case Crouching: StateFunction = &UPlayerMovementController::State_Crouch; break; case Crawling: StateFunction = &UPlayerMovementController::State_Crawl; break; case Pounding: StateFunction = &UPlayerMovementController::State_Pound; break; case PoundFlipping: StateFunction = &UPlayerMovementController::State_PoundFlip; break; case Diving: StateFunction = &UPlayerMovementController::State_Dive; break; case Dodging: StateFunction = &UPlayerMovementController::State_Dodge; break; default: break; } (this->*StateFunction)(EStateInput::INIT); //_Debug_PrintState(); //(this->*StateFunction)(EStateInput::TICK); // todo: should this be called here? } void UPlayerMovementController::NotifyStateChanged_Implementation(EMoveState NewState) { // ... } void UPlayerMovementController::NotifySetChanged_Implementation(EMoveSet NewSet) { NewMoveSet_Notified(NewSet); } void UPlayerMovementController::NotifyOnLanded_Implementation() { (this->*StateFunction)(EStateInput::EVENT_Landed); //Parent->JumpMaxCount = 1; } void UPlayerMovementController::NotifyOnJumped_Implementation() { (this->*StateFunction)(EStateInput::EVENT_Jumped); } void UPlayerMovementController::NotifyOnLaunched_Implementation() { (this->*StateFunction)(EStateInput::EVENT_Launched); } void UPlayerMovementController::NotifyHitMechanicFired_Implementation() { (this->*StateFunction)(EStateInput::EVENT_HitMechanic); } void UPlayerMovementController::NotifyCameraMechanicFired_Implementation() { (this->*StateFunction)(EStateInput::EVENT_CameraMechanic); } void UPlayerMovementController::NewState(void (UPlayerMovementController::* NewStateFunction)(EStateInput e)) { (this->*StateFunction)(EStateInput::END); StateFunction = NewStateFunction; (this->*StateFunction)(EStateInput::INIT); } void UPlayerMovementController::CallStateFunction(EStateInput Input) { (this->*StateFunction)(Input); } void UPlayerMovementController::_Debug_PrintState() { switch (Parent->GetMoveState()) { case Idle: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Idle")); break; case Walking: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Walking")); break; case Running: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Running")); break; case Jumping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Jumping")); break; case HighJumping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: HighJumping")); break; case SpinJumping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: SpinJumping")); break; case Falling: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Falling")); break; case Crouching: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Crouching")); break; case Crawling: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Crawling")); break; case Pounding: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Pounding")); break; case PoundFlipping: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: PoundFlipping")); break; case Diving: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Diving")); break; case Dodging: UE_LOG(LogTemp, Warning, TEXT("Current MoveState: Dodging")); break; default: break; } } void UPlayerMovementController::NewMoveSet(EMoveSet NewMoveSet) { Parent->_SetMoveSet(NewMoveSet); NewMoveSet_Notified(NewMoveSet); } void UPlayerMovementController::NewMoveSet_Notified(EMoveSet NewMoveSet) { (this->*StateFunction)(EStateInput::SET_END); this->MoveSet = NewMoveSet; (this->*StateFunction)(EStateInput::SET_INIT); } void UPlayerMovementController::Limited_Idle_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Idle); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (NormalMovement()) // If movement is being applied { NewState(EMoveState::Running); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crouching); return; case HIT_Pressed: // fire companion hit (prob in parent APlayer_Character) break; case END: break; default: break; } } void UPlayerMovementController::Limited_Run_Implementation(EStateInput input) { if (CommonActions(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Running); break; case TICK: if (Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Falling); return; } else if (!NormalMovement()) { NewState(EMoveState::Idle); return; } break; case JUMP_Pressed: NewState(EMoveState::Jumping); return; case CROUCH_Pressed: NewState(EMoveState::Crawling); break; default: break; } } void UPlayerMovementController::Limited_Jump_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (_Common_Limited_Jump_Actions(input)) { return; } //if (_RegularJump(input)) { return; } if (_JumpTransitionGuard(input)) { return; } switch (input) { case SET_INIT: Ref_CharacterMovement->JumpZVelocity = 300.f; break; case INIT: StandardJumpZVelocity = Ref_CharacterMovement->JumpZVelocity; Ref_CharacterMovement->JumpZVelocity = 300.f; Parent->Jump(); Parent->_SetMoveState(EMoveState::Jumping); if (!Parent->CanJump()) { NewState(EMoveState::Idle); return; } break; case TICK: if (!Ref_CharacterMovement->IsFalling() && (!JumpInputBuffered || !Parent->CanJump())) //todo: can you refactor this to EVENT_Landed? { NewState(EMoveState::Idle); return; } break; case JUMP_Released: Parent->StopJumping(); break; case EVENT_Landed: Parent->StopJumping(); break; case END: case SET_END: Ref_CharacterMovement->JumpZVelocity = StandardJumpZVelocity; break; default: break; } } bool UPlayerMovementController::_Common_Limited_Jump_Actions(EStateInput input) { if (__JumpBuffer(input)) { return true; } if (__JumpGravityScaling(input)) { return true; } if (__StickyLanding(input)) { return true; } switch (input) { case TICK: AirMovement(); break; case END: //Parent->JumpMaxCount = 1; //Parent->StopJumping(); break; case FLASH_Pressed: Parent->FireCameraMechanic(); break; default: break; } return false; } void UPlayerMovementController::Limited_Fall_Implementation(EStateInput input) { if (CommonOnLandedActions(input)) { return; } if (_Common_Limited_Jump_Actions(input)) { return; } if (_CoyoteTime(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Falling); break; case TICK: if (!Ref_CharacterMovement->IsFalling()) { NewState(EMoveState::Idle); return; } break; case END: break; default: break; } } void UPlayerMovementController::Limited_Crouch_Implementation(EStateInput input) { if (_CommonActions_Limited(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crouching); Parent->Crouch(); break; case TICK: if (NormalMovement()) { NewState(EMoveState::Crawling); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Idle); return; case END: //Parent->UnCrouch(); //todo: what if transitioning to crawl? does uncrouch, then crouch work? break; case RESET: Parent->UnCrouch(); break; default: break; } } bool UPlayerMovementController::_CommonActions_Limited(EStateInput input) { switch (input) { case FLASH_Pressed: Parent->FireCameraMechanic(); break; default: break; } return false; } void UPlayerMovementController::Limited_Crawl_Implementation(EStateInput input) { if (_CommonActions_Limited(input)) { return; } switch (input) { case INIT: Parent->_SetMoveState(EMoveState::Crawling); Parent->Crouch(); break; case TICK: if (!NormalMovement()) { NewState(EMoveState::Crouching); return; } break; case CROUCH_Released: Parent->UnCrouch(); NewState(EMoveState::Running); break; case END: //Parent->UnCrouch(); break; case RESET: Parent->UnCrouch(); break; default: break; } }
Living Design Documents
Methodology
Documentation needs to be up to date in order to be useful. In concert with our iterative playtest method, I incorporated design documentation that grows with the project.
Design decisions are proved via playtest.
After every playtest period, a new entry is created. This typically occurs at least once per sprint.
Feedback from each playtest is accrued and a list of issues is identified. The goal is to improve the feature by the next iteration. Team members comment on the issues list, and tasks are created for the next sprint period.
This is repeated for every new iteration.
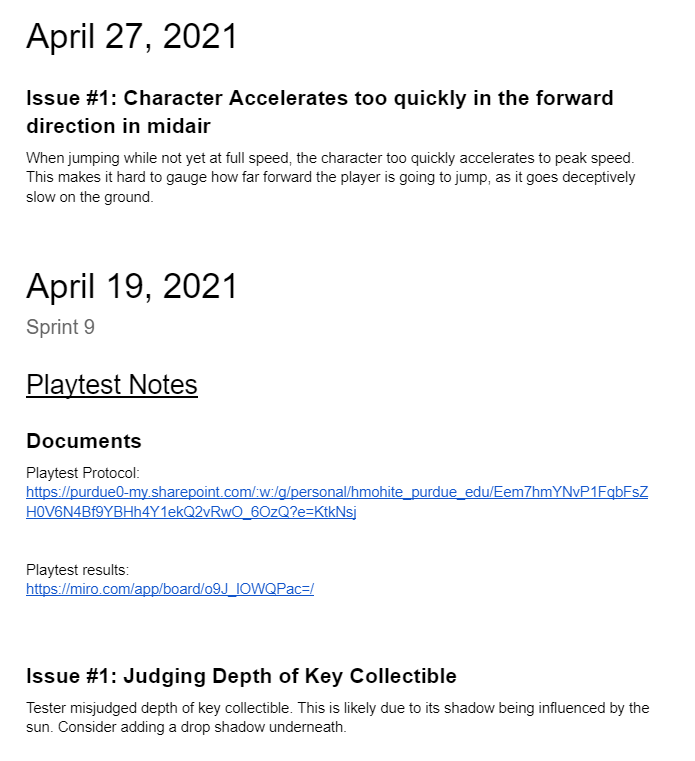
Methodology
Documentation needs to be up to date in order to be useful. In concert with our iterative playtest method, I incorporated design documentation that grows with the project.
Online tools allow team members to make in-line comments and suggestions, and all content links are set to update dynamically. This master document can be updated after design reviews, playtests, and at the end of sprints as needed.
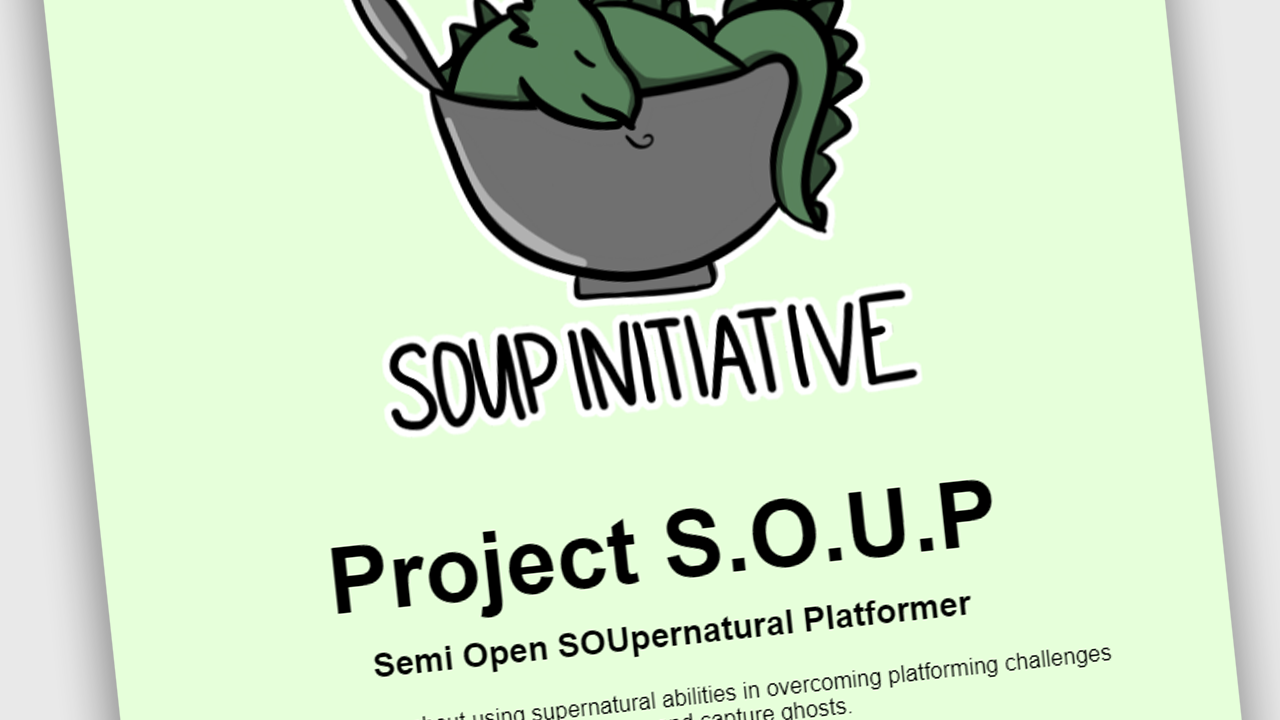
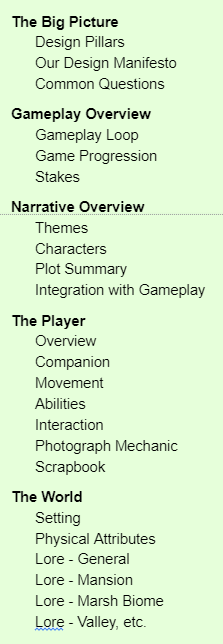
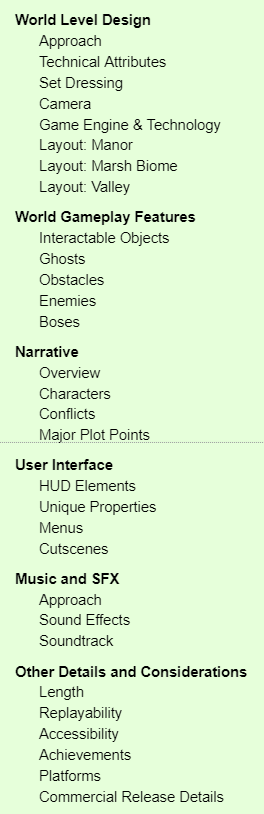